MODBUS registers and nodes.
Application Description:
This example shows the use of internal MODBUS registers on the Universal-Robots and also using external MODBUS nodes.
The application uses the MODBUS server running at port 502 on the Universal-Robots. The MODBUS server has a range of register available with dedicated content and register 128 to 255 is for general purpose use.
A list of the MODBUS and register can be found at this link UR Modbus page
The commands are send from a external host with Python code.
Function description:
The nodes used are Beckhoff model
BK 9050 = Modbus Controller with Ethernet port for IP configuration.
KL 1408 = Modbus 8 port input module.
KL 2408 = Modbus 8 port output module.
KL 9010 = Modbus End terminator.
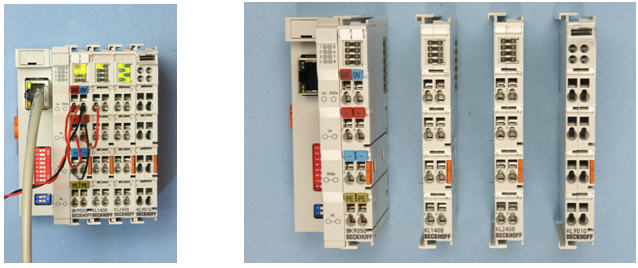
The IP address of the Modbus node has to be defined in the Robot configuration.
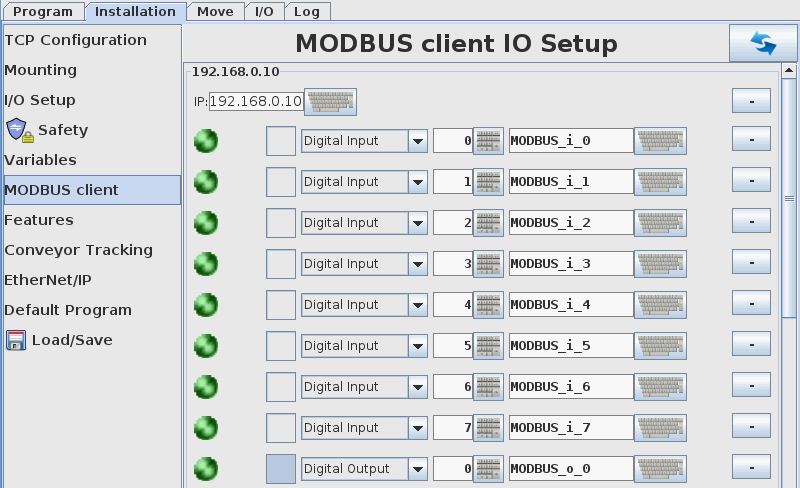
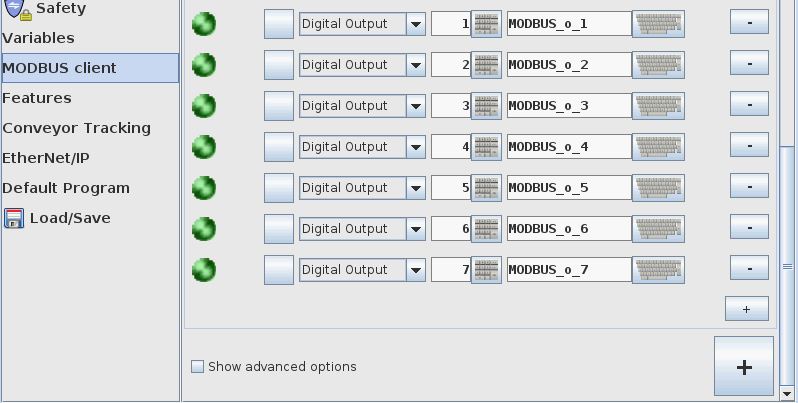
I/O table Inputs:
Variable Table:
var_1 = Modbus signal from external Modbus node (bit 0)
var_2 = Value of register 128 (set by remote host)
Electrical diagrams.
Program description:
As this program is for testing the MODBUS interface the robot program has only one waypoint.
Then the status of MODBUS node input 0 is read into variable var_1. Followed by reading the value register 128.
Then register 129 is toggled between the value 05 and value 00 with a 1 sec interval.
In parallel program Thread_1 the MODBUS output 0 is toggled between 1 and 0.
In parallel program Thread_2 the MODBUS output 4 is set same value as MODBUS input 0.
Program code:
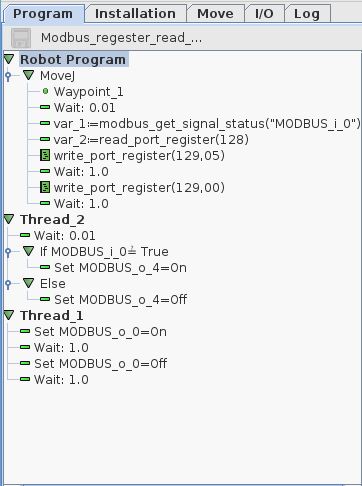
Host program in Python code.
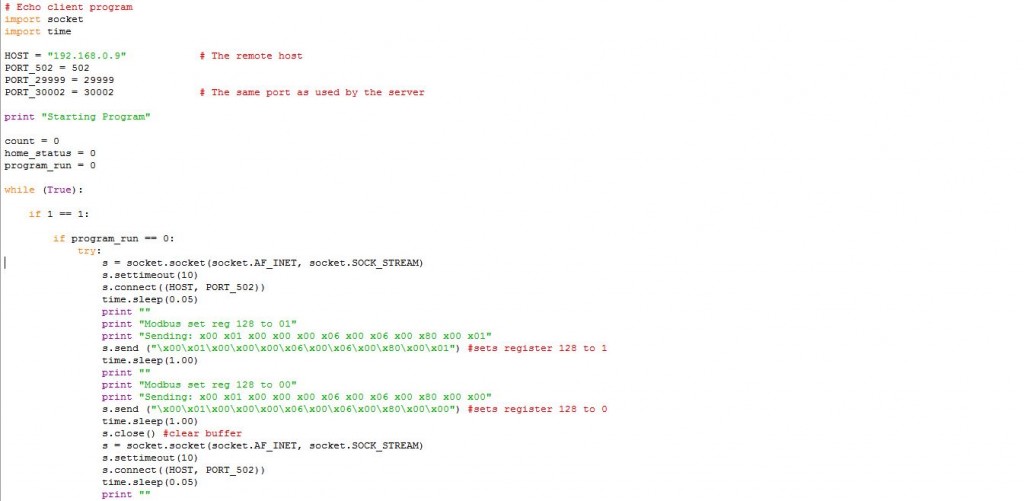
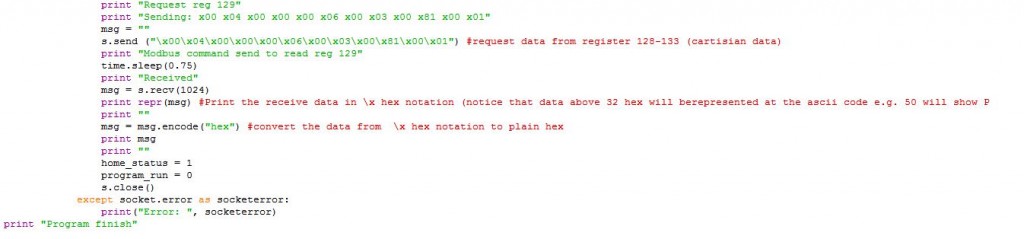
# Echo client program import socket import time HOST = "192.168.0.9" # The remote host PORT_502 = 502 PORT_29999 = 29999 PORT_30002 = 30002 # The same port as used by the server print "Starting Program" count = 0 home_status = 0 program_run = 0 while (True): if 1 == 1: if program_run == 0: try: s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.settimeout(10) s.connect((HOST, PORT_502)) time.sleep(0.05) print "" print "Modbus set reg 128 to 01" print "Sending: x00 x01 x00 x00 x00 x06 x00 x06 x00 x80 x00 x01" s.send ("\x00\x01\x00\x00\x00\x06\x00\x06\x00\x80\x00\x01") #sets register 128 to 1 time.sleep(1.00) print "" print "Modbus set reg 128 to 00" print "Sending: x00 x01 x00 x00 x00 x06 x00 x06 x00 x80 x00 x00" s.send ("\x00\x01\x00\x00\x00\x06\x00\x06\x00\x80\x00\x00") #sets register 128 to 0 time.sleep(1.00) s.close() #clear buffer s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.settimeout(10) s.connect((HOST, PORT_502)) time.sleep(0.05) print "" print "Request reg 129" print "Sending: x00 x04 x00 x00 x00 x06 x00 x03 x00 x81 x00 x01" msg = "" s.send ("\x00\x04\x00\x00\x00\x06\x00\x03\x00\x81\x00\x01") #request data from register 128-133 (cartisian data) print "Modbus command send to read reg 129" time.sleep(0.75) print "Received" msg = s.recv(1024) print repr(msg) #Print the receive data in \x hex notation (notice that data above 32 hex will berepresented at the ascii code e.g. 50 will show P print "" msg = msg.encode("hex") #convert the data from \x hex notation to plain hex print msg print "" home_status = 1 program_run = 0 s.close() except socket.error as socketerror: print("Error: ", socketerror) print "Program finish"
Program run:
The var_1 is getting the value of True and False according to the settings of input 0 on the external MODBUS node.
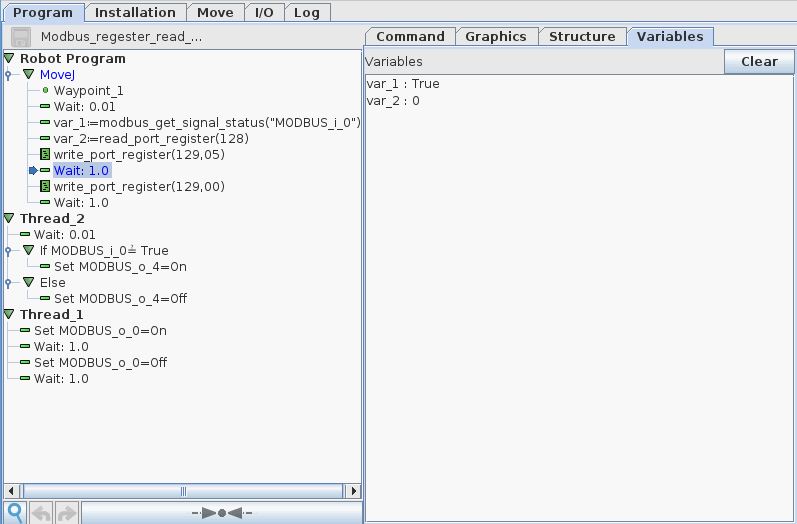
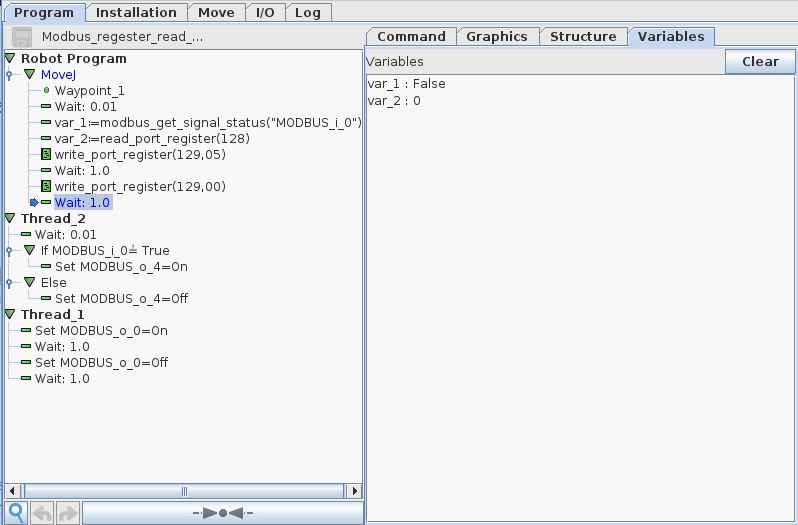
The MODBUS output 0 is toggleing between True and False with a 1 second interval as controlled by Thread_1
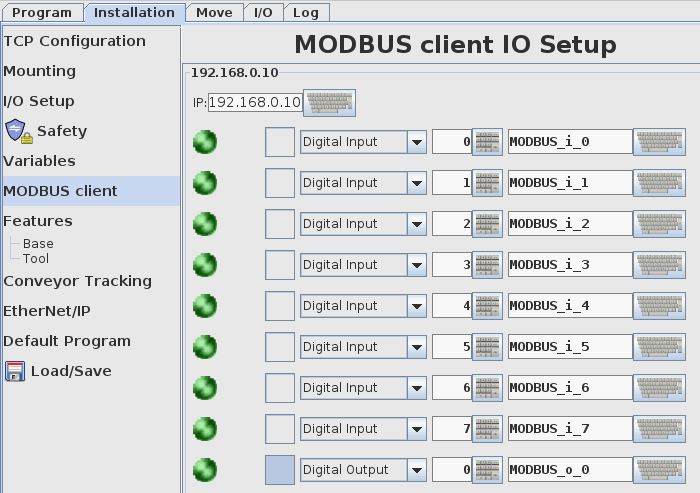
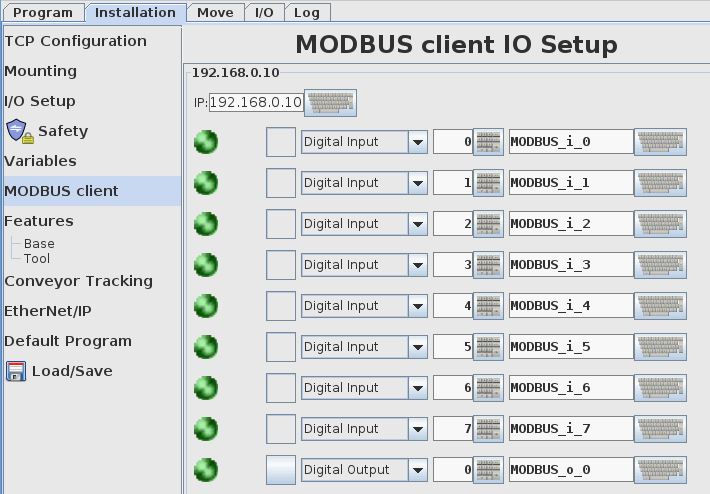
Data received on the external host with description below.
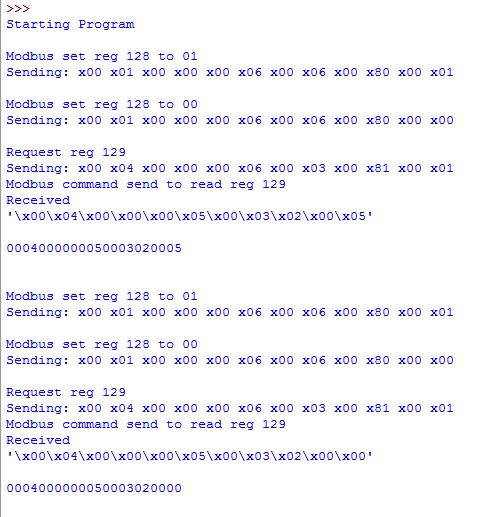
Notes:
Data exchange using the Python program:
Modbus Data send to UR to write UR register.
First request to write.
Data send
\x00\x01\x00\x00\x00\x06\x00\x06\x00\x80\x00\x01
Which is the same as
00 01 00 00 00 06 00 06 00 80 00 01
Meaning:
00 01 is the sequence number Modbus TCP follows (Sequence no = Transaction identifier (serial no)
00 00 is protocol indentifier
00 06 messages length – means 6 bytes will follow
00 Unit identifier – or slave address
06 is the function code for write
00 80 The address of the first register to set (register 80hex = 128 dec).
00 01 The value to set into register 128 (set value to 1)
Second request to write.
Data send.
\x00\x01\x00\x00\x00\x06\x00\x06\x00\x80\x00\x00
Which is the same as
00 01 00 00 00 06 00 06 00 80 00 00
Meaning:
00 01 is the sequence number Modbus TCP follows (Sequence no = Transaction identifier (serial no)
00 00 is protocol indentifier
00 06 messages length – means 6 bytes will follow
00 Unit identifier – or slave address
06 is the function code for write
00 80 The address of the first register to set (register 80hex = 128 dec).
00 00 The value to set into register 128 (set value to 0)
Modbus Data send to UR to read UR register.
\x00\x04\x00\x00\x00\x06\x00\x03\x00\x81\x00\x01
Which is the same as
00 04 00 00 00 06 00 03 00 81 00 01
Meaning:
00 04 is the sequence number Modbus TCP follows (Sequence no = Transaction identifier (serial no)
00 00 is protocol indentifier
00 06 messages length – means 6 bytes will follow
00 Unit identifier – or slave address
03 is the function code for read
00 81 The data address of the first register requested (register 81hex = 129 dec).
00 01 The total number of register requested
Data received from UR
First poll received:
0004000000050003020000
Meaning:
00 04 is the sequence number that Modbus TCP follow (same as in the request send i.e. replied to)
00 00 Protocol identifier
00 05 messages length – means 5 bytes will follow
03 was the function code requested
02 registeres are read
00 00 is data value received.
Another pool received.
0004000000050003020005
Meaning:
00 04 is the sequence number that Modbus TCP follow (same as in the request send i.e. replied to)
00 00 Protocol identifier
00 05 messages length – means 5 bytes will follow
03 was the function code requested
02 registeres are read
00 05 is data value received.
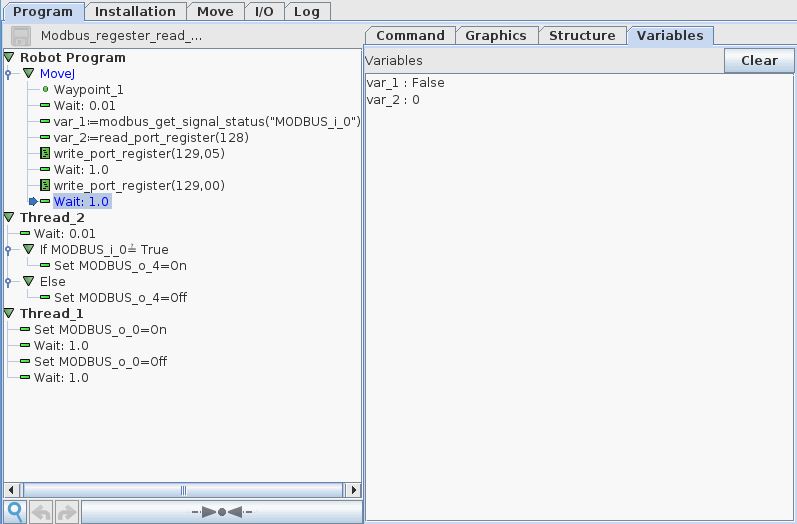
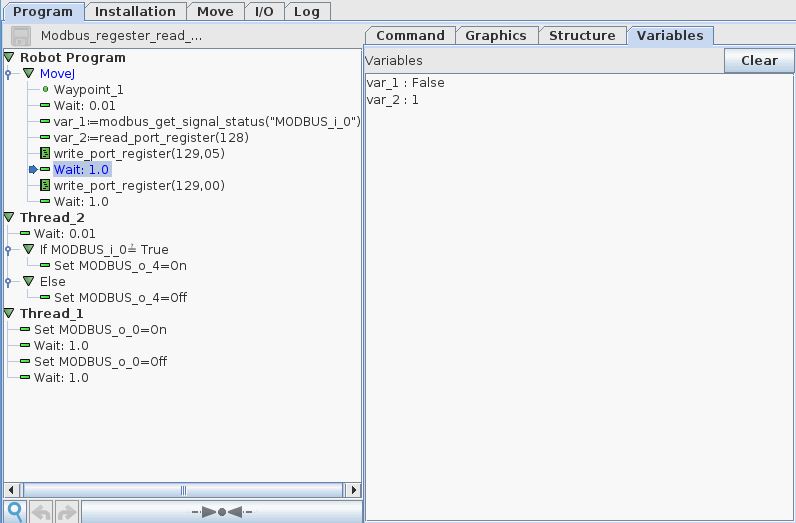
Disclaimer: While the Zacobria Pte. Ltd. believes that information and guidance provided is correct, parties must rely upon their skill and judgement when making use of them. Zacobria Pte. Ltd. assumes no liability for loss or damage caused by error or omission, whether such an error or omission is the result of negligence or any other cause. Where reference is made to legislation it is not to be considered as legal advice. Any and all such liability is disclaimed.
If you need specific advice (for example, medical, legal, financial or risk management), please seek a professional who is licensed or knowledgeable in that area.
Author:
By Zacobria Lars Skovsgaard
Accredited 2015-2018 Universal Robots support Centre and Forum.