UR Programming Functions:
Application Description:
This article shows how it is possible to make functions in script files that can be embedded into a UR program.
Example 1: Function for Control Outputs from a local script file.
Example 2: Function with parameter transfer.
Example 3: Function with parameter transfer and make calculation and return result.
Example 4: Function with move command and calculate new position for move.
Example 5: Function with If condition.
Example 6: Function that combine all of the above 5 examples.
Example 7: Function with 2 lists of poses – (Each list contain 6 poses i.e. a total of 12 poses).
A function is typically a small piece of code that performs a calculation routine or can be programs lines that are often used in the main program.
Some benefits of using the method of Functions is that such script files can be maintained offline, but needs to be transferred and loaded into the Polyscope program (compiled) to take effect.
Another benefit is that the main Polyscope program can be fewer lines.
However a disadvantage might be that the program is more difficult to troubleshoot because the UR will only show an error for the Script – not where in the script the error is. So some evaluation for Pros and Cons needs to be performed.
As describe in other articles on this forum Script commands and Script files can be used in various ways with a Universal-Robots – either send from an external device or used locally on the UR – or a combination of that.
Note: Variables will not work when sending as raw script commands because variables (and other programming technique like loop – If-Else etc) needs to be controlled either by the external host program (e.g. a Python program) – or within a Polyscope program. Such variable cannot be left in between a host program and a Polyscope program – there is no controller in between.
To send variables – make loops – use If-Else and to get informations back from the robot then the client-server method can be considered.
UR Script: Client-Server example.
A function might not be ideally transferred from an external device via port 30001 – 30002- 30002 and to expect return data back to the external device. Other approaches can be used if this is the goal – like Client-Server situation and use of the UR MODBUS server on Port 502.
Other articles on this forum describe how it is possible to send raw Script commands from an external device e.g. a PC to the robot on Port 30001 – 30002 and 30003 – and is considered as a kind of “One Way” commands – meaning it is not ideal to ask for data back with this method. The robot does automatically send data back from Port 30002 which is a kind of fixed Matlab data.
Instead a function is best and most ideally used locally on the UR and the function code will be embedded into the Polyscope program. The script file can be prepared on an external device for example if the robot is already in production and then when the robot is idle and available – then the script file can be transferred to the UR and then manually embedded into the Polyscope program.
Function description:
A function program typically looks like this
def myProgram() Some script code…….. “ “ end
Although it is possible to send code that looks like a function to Port 30001– 30002 – 30003, and the robot will execute this, Something like this below can be send to Port 30002 and it will be executed.
def Move(): followed by a \n (newline) set_digital_out(1,True) followed by a \n (newline) movel(p[0.0,0.3,0.3,0.0,3.14,0.0], a=1, v=1) followed by a \n (newline) set_digital_out(1,False) followed by a \n (newline) end followed by a \n (newline)
Turning Output 1 on then move the robot to position X=0, Y=300mm, Z=300mm, Rx=0, Ry = 3.14, Rz = 0 and the turn Output 1 off.
But with this method is not ideal to read Inputs or use flow controls likes “IF” or “While” or “sleep(x.x) with this method. For example a command like sleep(5.0) will not result in a 5 second delay. This is because every new line will be executed immediately. Therefore if the previous command is not finished – then this previous command will be terminated and the new command will be executed.
And flow controls like “If” and “While” also does not execute when they are send to port 30002 from an external device. And similar a “sleep(10) (wait 10 seconds) will not be executed if send through port 30002.
So – if the goal is to read data back from variables or inputs and use of flow controls (If and While statements) then another approach can be used like a UR Client-Server situation – or read data from the UR robot MODBUS registers via Port 502
Instead this article will show some example on how functions can be used locally on the UR robot and then be embedded into the Polyscope program.
It is important to notice that a function becomes an embedded part of the Polycope program (compiled together). So if the function file has been changed – this file needs to be loaded into the Polyscope program again (compiled together) in order for the changes in the function to take effect.
Program description:
The following 6 examples show the use of functions locally on the UR robot.
Program code:
Example 1: Function for Control Outputs from a local script file.
A script file is create in Notepad on a PC. When the script file is embedded into the Polyscope and called from the main program the code in the script file will turn Output 1 On and OFF with a wait of 1 second interval in between.
This file is transferred to the robot or it can be loaded directly from a USB drive inserted into the UR robot.
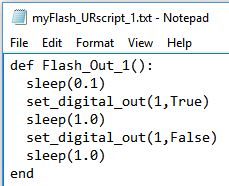
def Flash_Out_1(): sleep(0.1) set_digital_out(1,True) sleep(1.0) set_digital_out(1,False) sleep(1.0) end
Polyscope program is created.
A BeforeStart sequence is inserted.
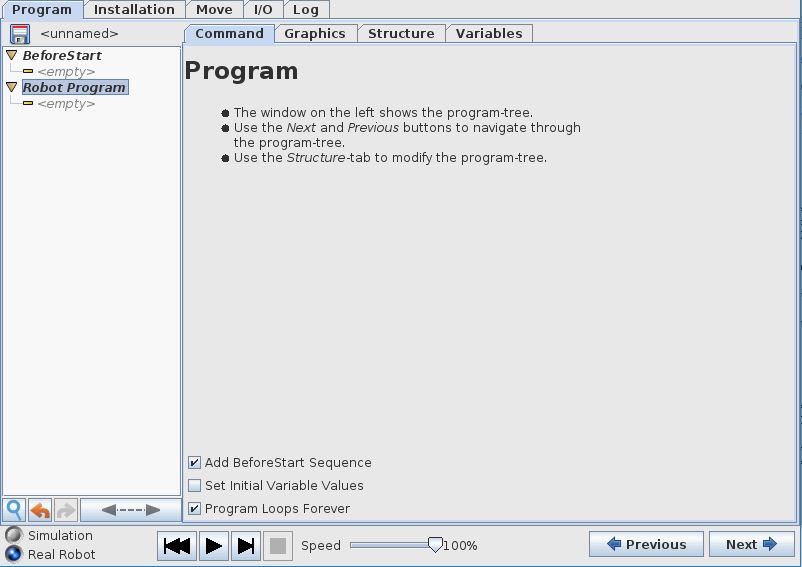
In the BeforeStart sequence a “Script entry” is selected from the Advances menu.
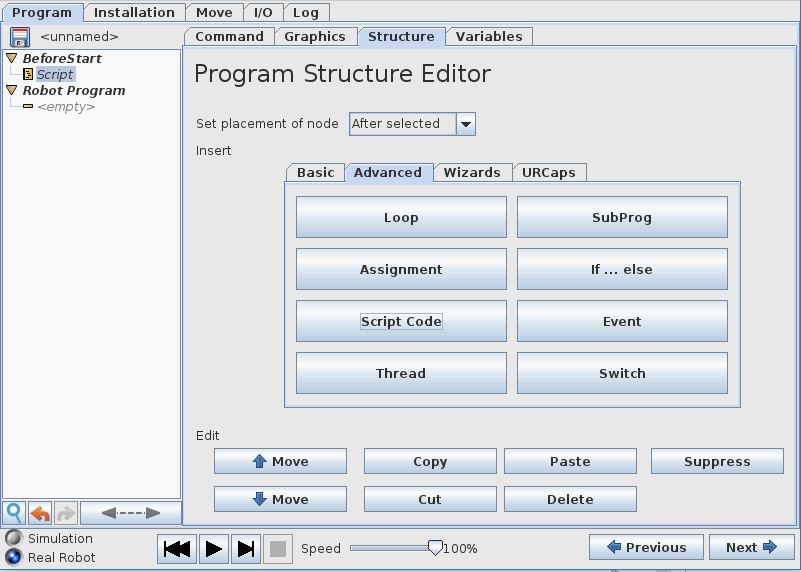
From the Command tab “File” is selected.
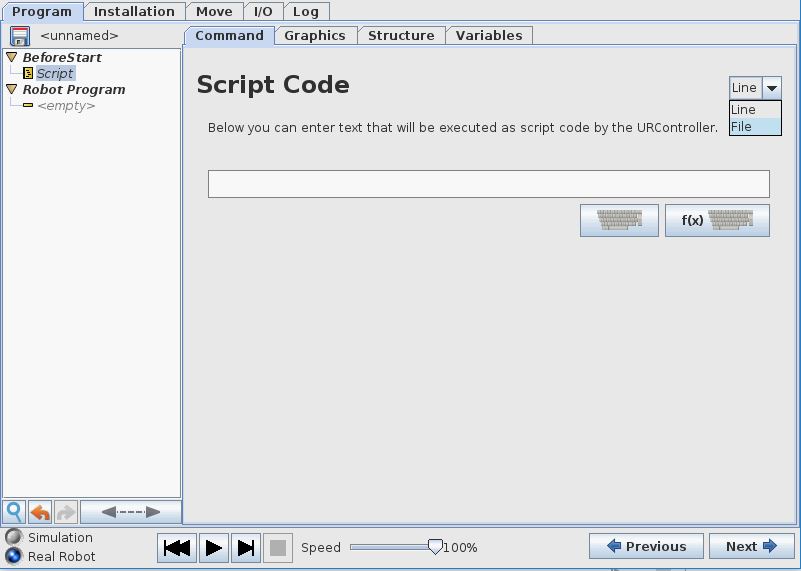
To enter the editor and to import the script file “Edit” is selected.
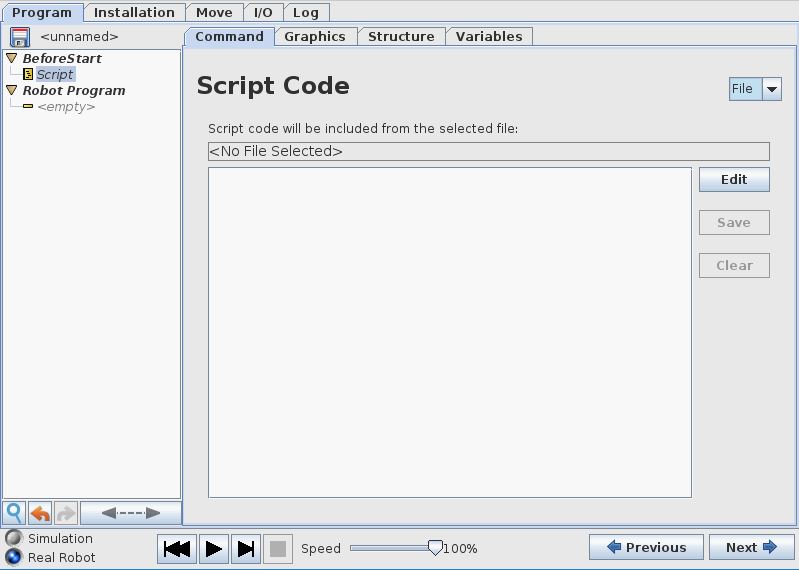
An editor opens up.
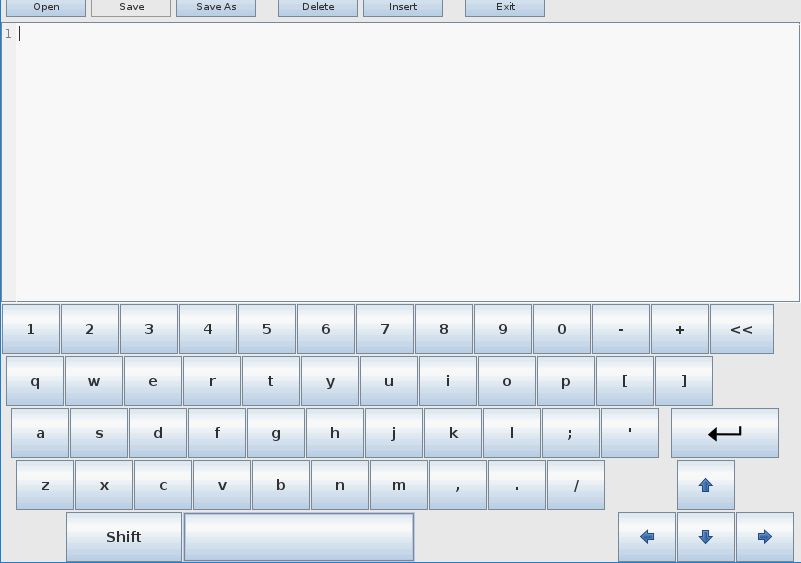
To import the script file “Open” is selected and the script file is pointed out.
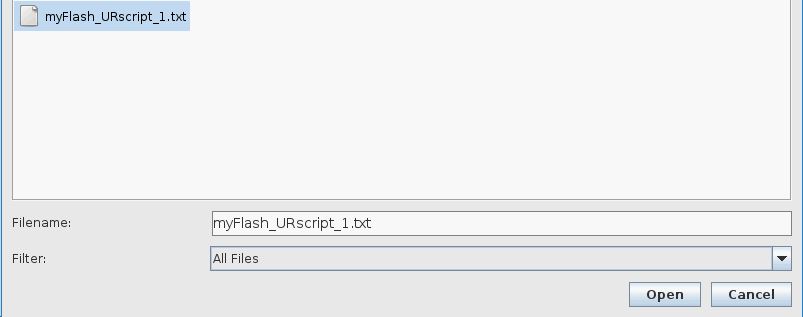
The contents of the script file is now in the editor on the robot.
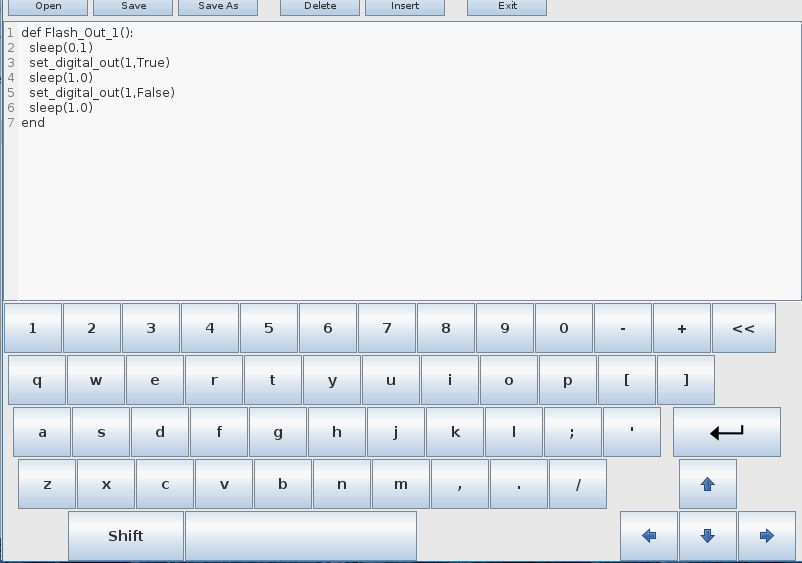
After selecting “Exit” in the editor – the script file is now inserted into the Polyscope program.
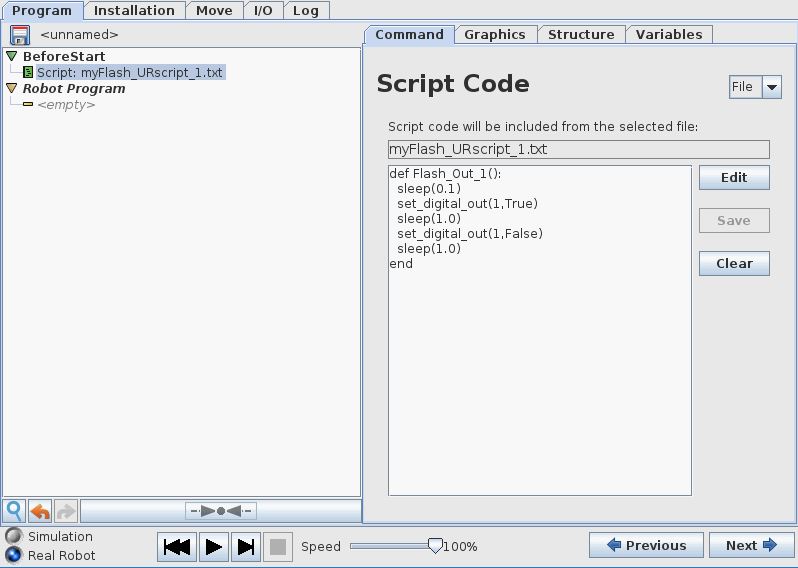
A small wait is inserted in order to prevent “infinite loop error”.
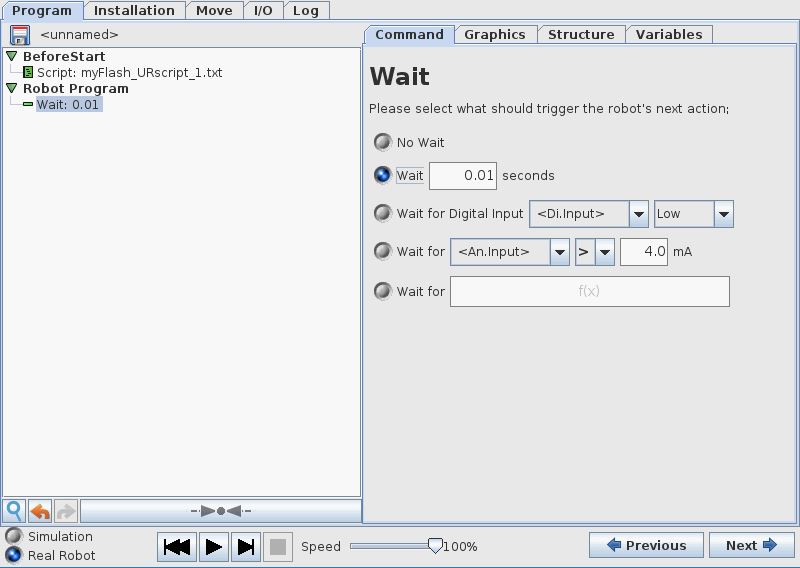
In order to call the script file from the main Polyscope program – a “Script entry” is selected from the Advanced menu.
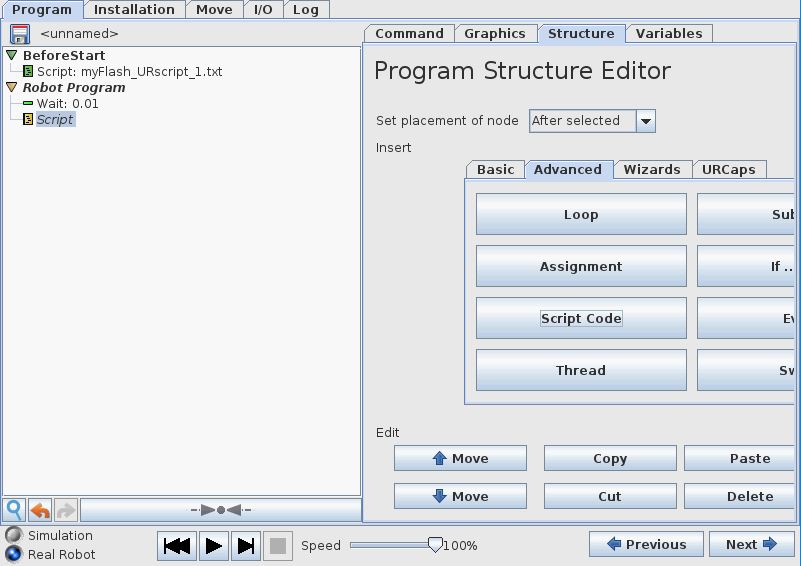
This time the “Line” entry for a single line script command is selected.
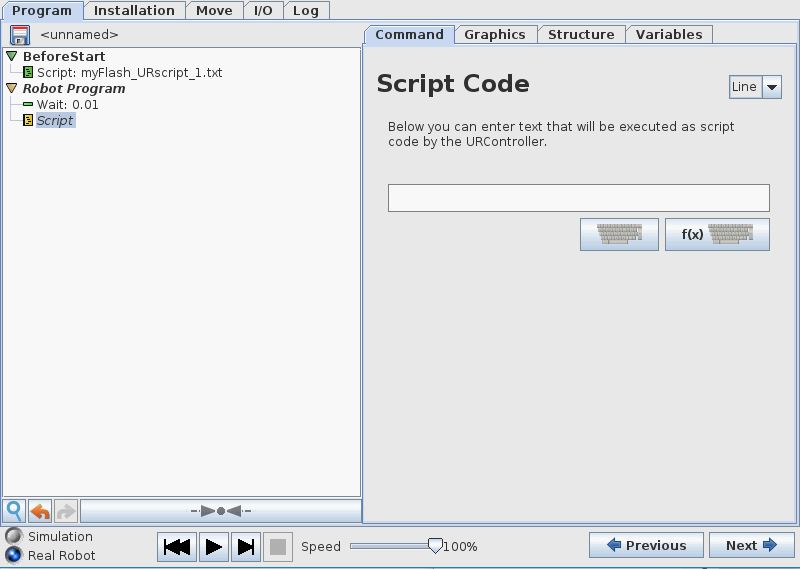
The script file is called with the command “Flash_Out_1()” because this is the name that is defined in the script file for this function.
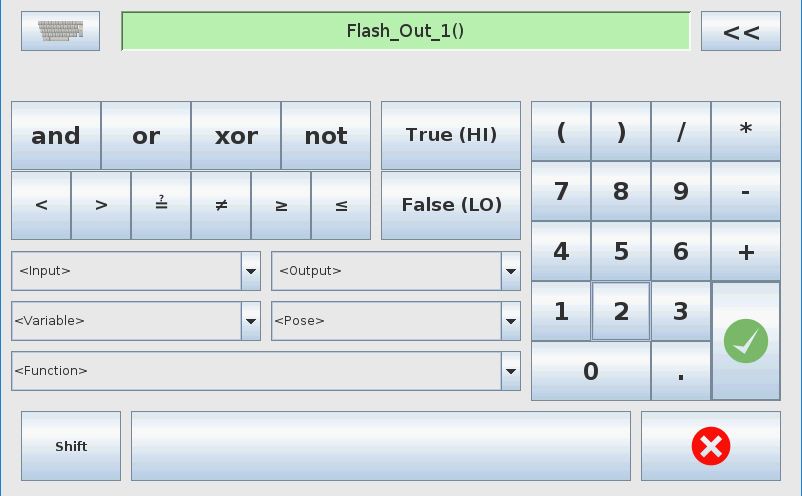
The call the the function “Flash_Out_1” In the Polyscope program.
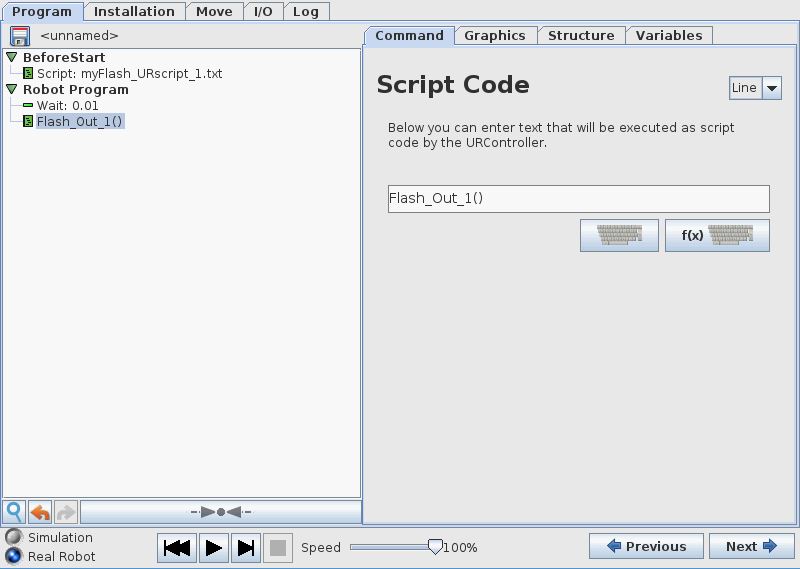
The program file is saved.
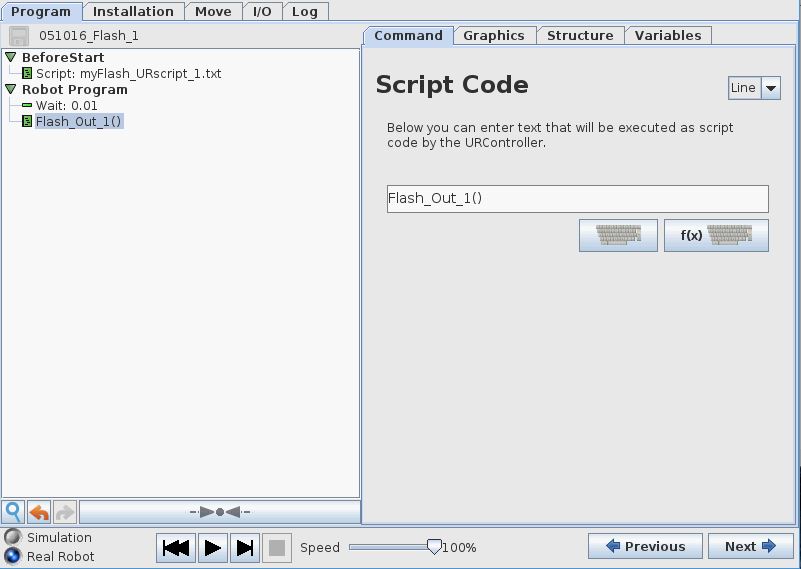
Program run:
To run the program the “Play” is pressed.
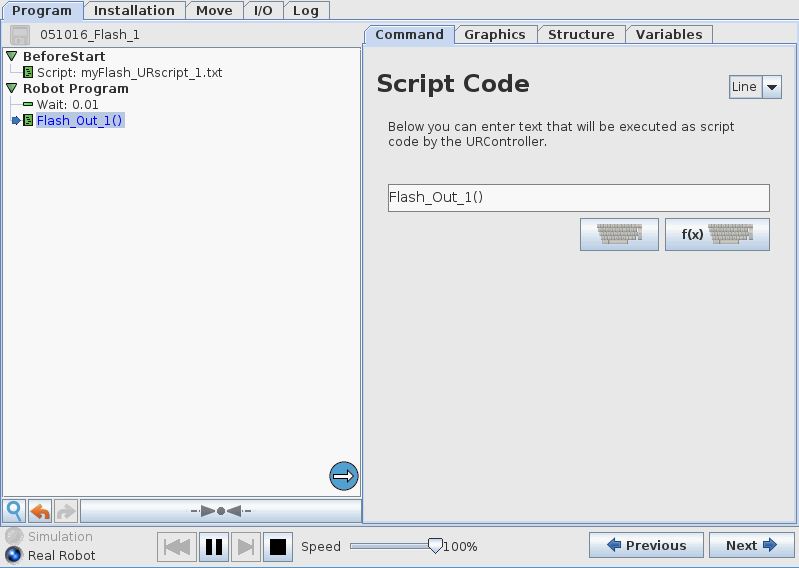
Digital Output 1 is tuned On and Off according to the code in the script file.
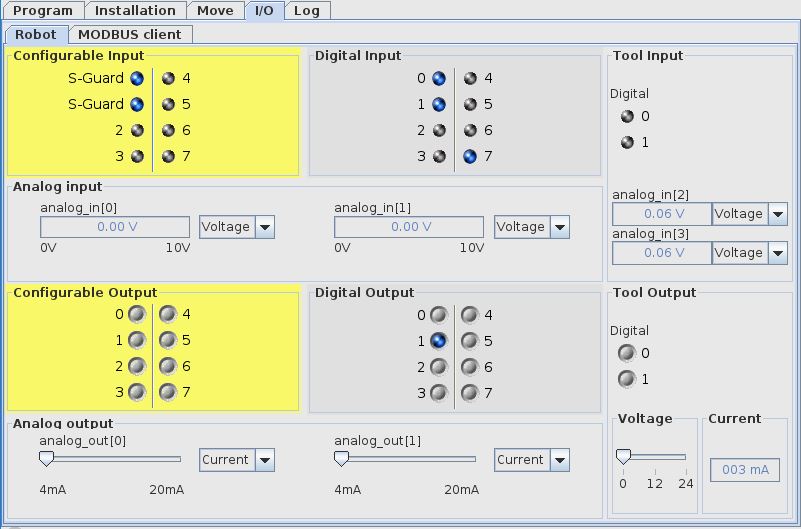
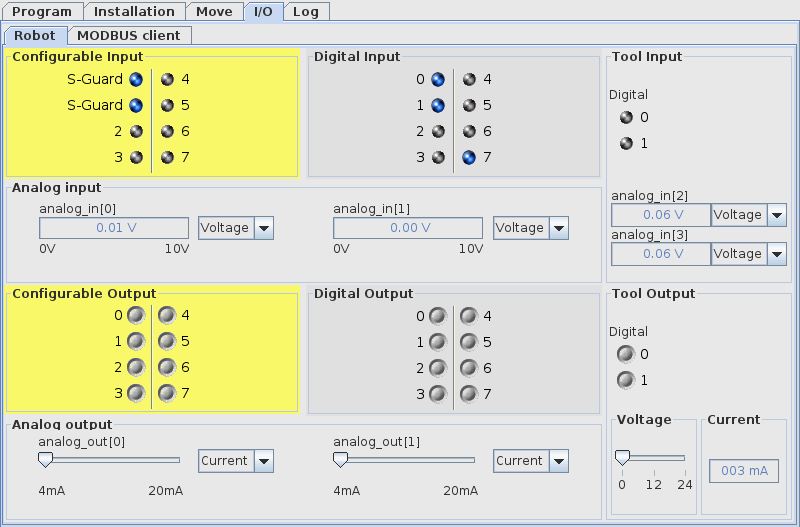
The code in the script file only show as one line in the main program, but behind the code in the script file is being executed.
Example 2: Function with parameter transfer.
A script file is create in Notepad on a PC. When the script file is embedded into the Polyscope and called from the main program the code in the script file will make a pause based on the value transferred into the function by the variable “counter”.
This file is transferred to the robot or it can be loaded directly from a USB drive inserted into the UR robot.
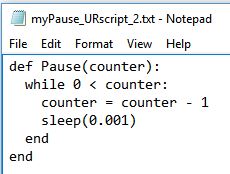
def Pause(counter): while 0 < counter: counter = counter - 1 sleep(0.001) end end
Polyscope program is created.
A BeforeStart sequence is inserted where the script file is loaded into the main Polyscope program.
In the main program Digital Output 1 is set to high. And the variable in this example is set to the value 1000.
The function “Pause” is called with the parameter value of variable var_1. The function provied a pause until var_1 is counted down from 1000 to 0.
Then the Digital Output is set to low and a new pause is inserted. So this program toggles Digital Output 1 on/off.
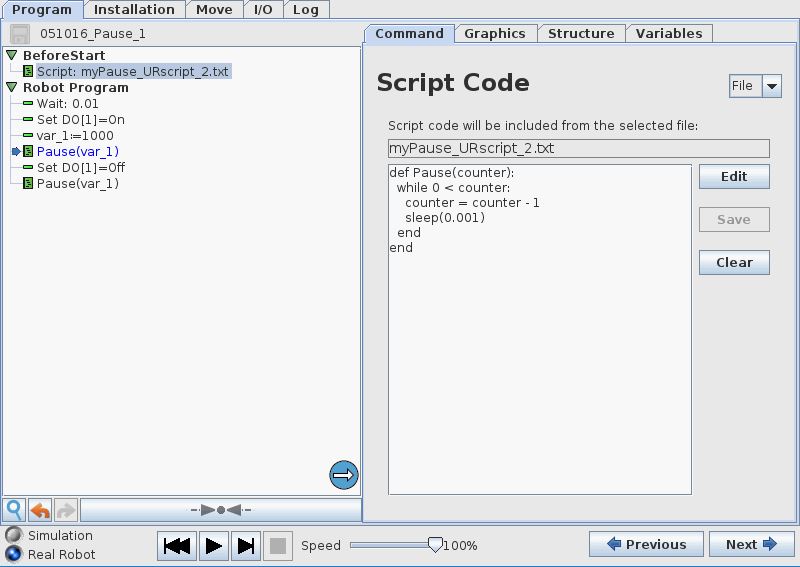
Program run:
Digital Output 1 is observed from the I/O menu and Digital Output toggles on/off.
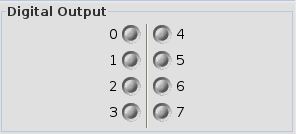
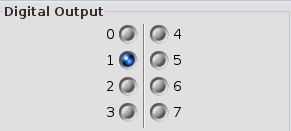
Example 3: Function with parameter transfer and make calculation and return result.
A script file is create in Notepad on a PC. When the script file is embedded into the Polyscope and called from the main program the code in the script file will calculate adding the local variable a and b – and also subtract a and b. The variable d is made global so it can be used directly in the main program. The variable c is local in the function and c is returned into the variable from where it was transferred as a parameter – now with a new value based on the calculation in the function.
This file is transferred to the robot or it can be loaded directly from a USB drive inserted into the UR robot.
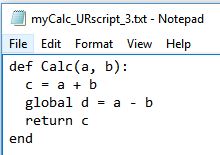
def Calc(a, b): c = a + b global d = a - b return c end
Polyscope program is created.
A BeforeStart sequence is inserted where the script file is loaded into the main Polyscope program.
In the main program the variable var_1 is set to 1 and the variable var_2 is set to 2.
The variable “Result” is set to the result of the calculation in the function by calling “Calc” with the var_1 and var_2 as the two parameters which are transferred into the function and their value is used for the calculation.
var_3 is set to the value of the variable Result.
var_4 is set to the value of the global variable d.
In this example the program is Halted just to be able to verify the variable values before a new program run.
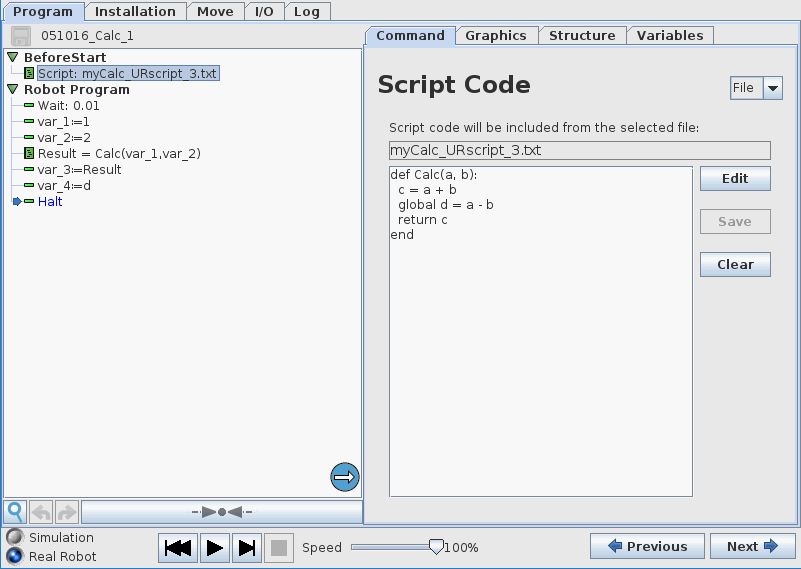
Program run:
The variables are confirmed in the Variables tab window.
var_1 and var_2 has their original value.
var_3 is the result of a + b.
var_4 is the result of a – b.
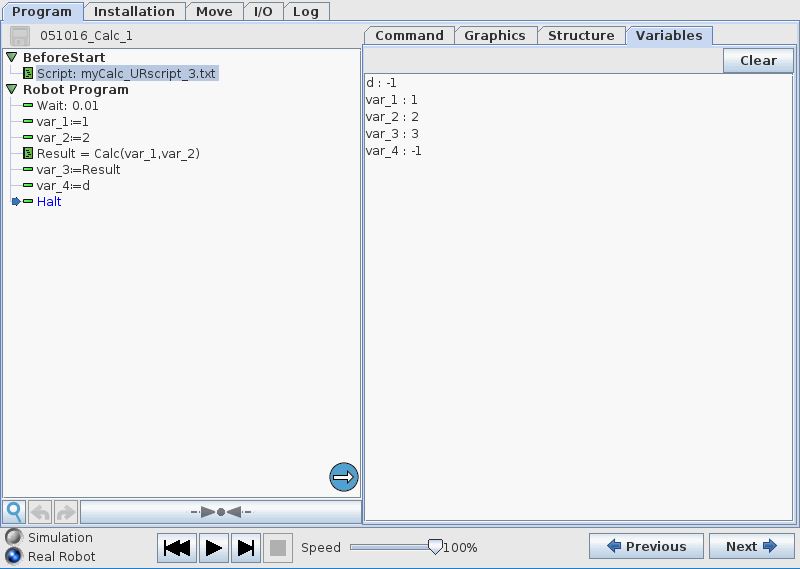
Example 4: Function with move command and calculate new position for move.
A script file is create in Notepad on a PC. When the script file is embedded into the Polyscope and called from the main program the code in the script file will move to the position based on the transferred values from the main program. Then wait 1 second and calculate new values for x, y and z. And then move to the new calculated position.
This file is transferred to the robot or it can be loaded directly from a USB drive inserted into the UR robot.
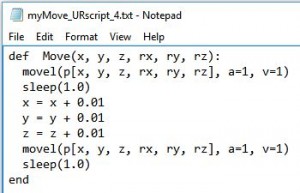
def Move(x, y, z, rx, ry, rz): movel(p[x, y, z, rx, ry, rz], a=1, v=1) sleep(1.0) x = x + 0.01 y = y + 0.01 z = z + 0.01 movel(p[x, y, z, rx, ry, rz], a=1, v=1) sleep(1.0) end
Polyscope program is created.
A BeforeStart sequence is inserted where the script file is loaded into the main Polyscope program.
In the main program the variable x is set to 0.0, variable y is set to 0.3, variable z is set to 0.3, variable Rx is set to 0.0, variable Ry is set to 3.14 and variable Rz is set to 0.0.
The function “Move” is called with the 6 variables above as paremetrs which are therefore transferred into the function.
In the function the robot first moves to the position of x, y, z, Rx, Ry, Rz.
The the function wait 1 second.
The variables x, y and z are added by the value 0.01 (1 cm).
The the robot moves to the new calculated position.
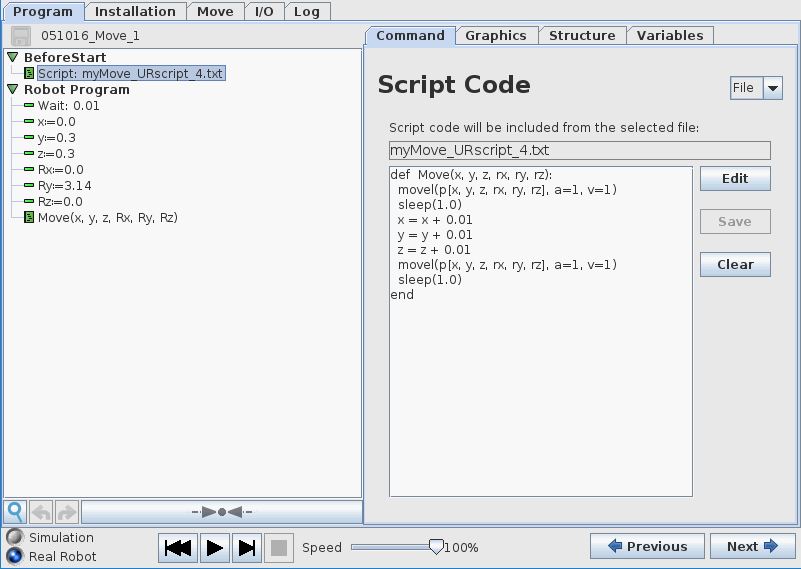
Program run:
The robot position is observed as it moves between the two positions.
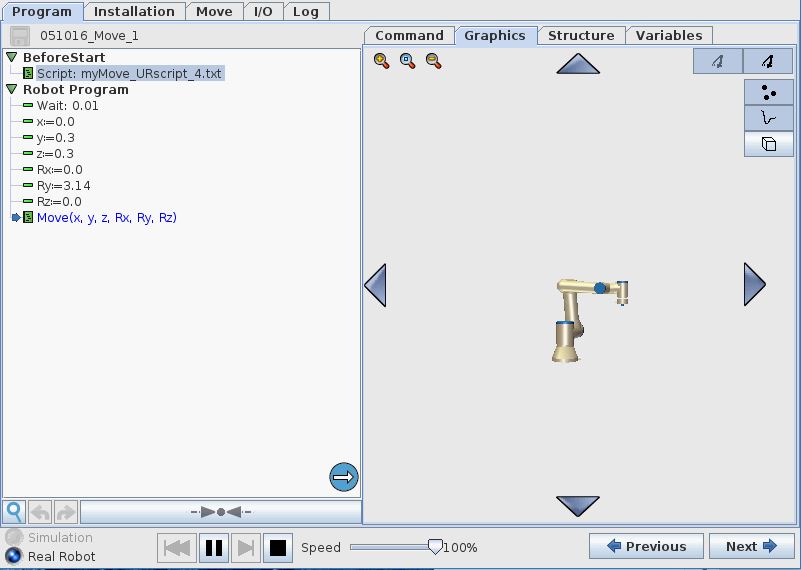
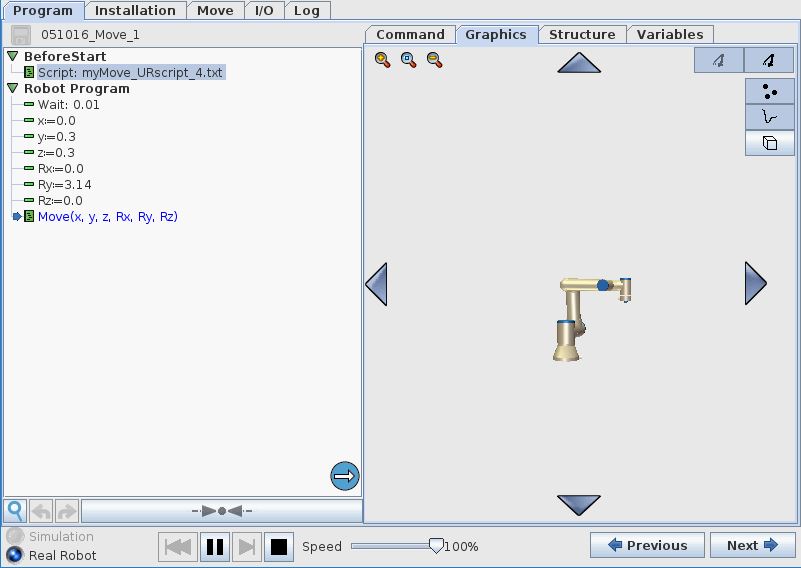
Example 5: Function with If condition.
A script file is create in Notepad on a PC. When the script file is embedded into the Polyscope and called from the main program the code in the script file will make a check on the status of Digital Input 0. If the input is True the robot will move to one position and if the input is False the robot will move to another position.
Notice; the value of x is 0.2 or -0.2 based on the status of input 0.
This file is transferred to the robot or it can be loaded directly from a USB drive inserted into the UR robot.
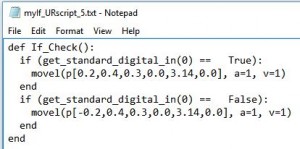
def If_Check(): if (get_standard_digital_in(0) == True): movel(p[0.2,0.4,0.3,0.0,3.14,0.0], a=1, v=1) end if (get_standard_digital_in(0) == False): movel(p[-0.2,0.4,0.3,0.0,3.14,0.0], a=1, v=1) end end
Polyscope program is created.
A BeforeStart sequence is inserted where the script file is loaded into the main Polyscope program.
A small wait is inserted in order to avoid “infinite loop” error messages.
The function “If_Check” is called which moves the robot between two position based on the status of the Digital Input 0.
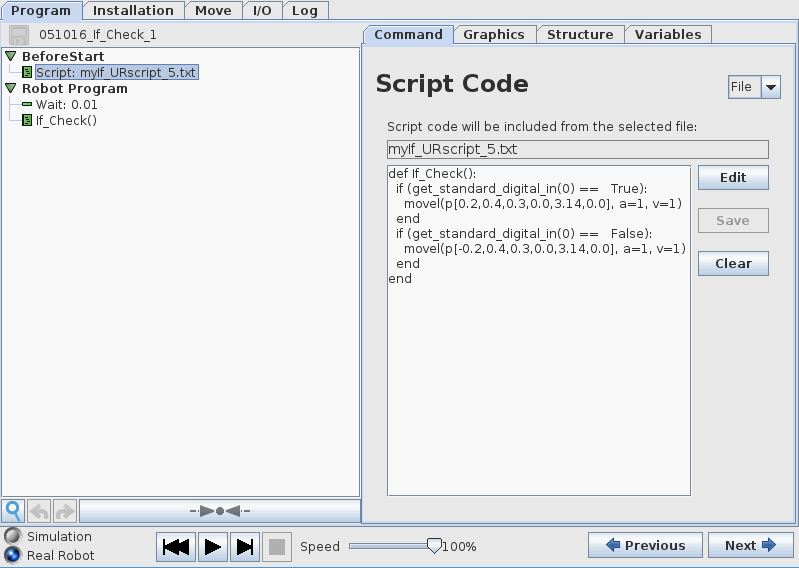
Program run:
Digital input is set to True.
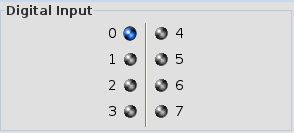
Robot moves to one side.
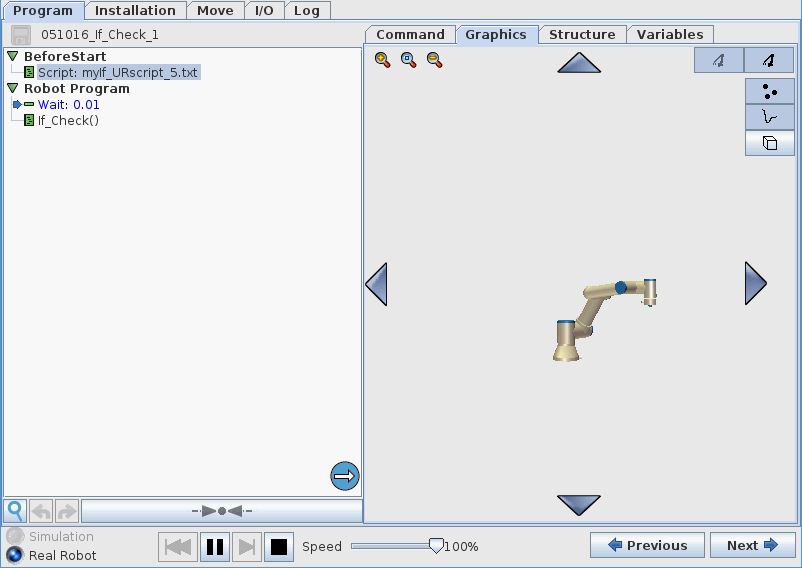
Digital input is set to False.
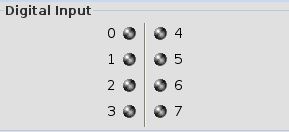
Robot moves to the other side.
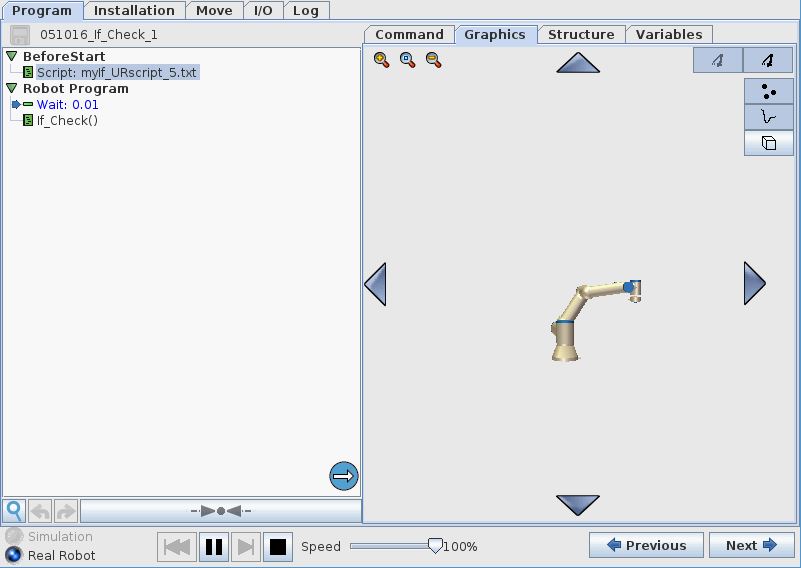
Example 6: Function that combine all of the above 5 examples.
A script file is create in Notepad on a PC. When the script file is embedded into the Polyscope and called from the main program the code in the script file will turn Output 1 On and OFF with a wait of 1 second interval in between.
Then make a pause based on the value transferred into the function by the variable “counter”.
Then calculate adding the local variable a and b – and also subtract a and b. The variable d is made global so it can be used directly in the main program. The variable c is local in the function and c is returned into the variable from where it was transferred as a parameter – now with a new value based on the calculation in the function.
Then move to the position based on the transferred values from the main program. Then wait 1 second and calculate new values for x, y and z. And then move to the new calculated position.
Then make a check on the status of Digital Input 0. If the input is True the robot will move to one position and if the input is False the robot will move to another position.
Notice; the value of x is 0.2 or -0.2 based on the status of input 0.
This file is transferred to the robot or it can be loaded directly from a USB drive inserted into the UR robot.
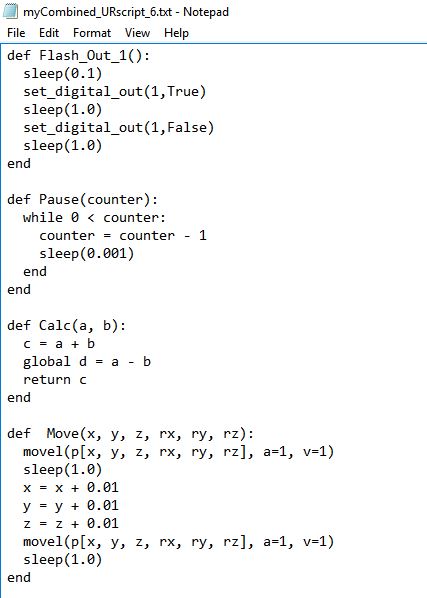
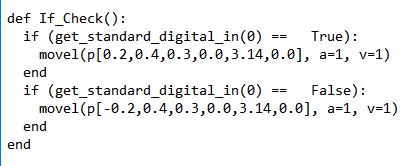
def Flash_Out_1(): sleep(0.1) set_digital_out(1,True) sleep(1.0) set_digital_out(1,False) sleep(1.0) end
def Pause(counter): while 0 < counter: counter = counter - 1 sleep(0.001) end end
def Calc(a, b): c = a + b global d = a - b return c end
def Move(x, y, z, rx, ry, rz): movel(p[x, y, z, rx, ry, rz], a=1, v=1) sleep(1.0) x = x + 0.01 y = y + 0.01 z = z + 0.01 movel(p[x, y, z, rx, ry, rz], a=1, v=1) sleep(1.0) end
def If_Check(): if (get_standard_digital_in(0) == True): movel(p[0.2,0.4,0.3,0.0,3.14,0.0], a=1, v=1) end if (get_standard_digital_in(0) == False): movel(p[-0.2,0.4,0.3,0.0,3.14,0.0], a=1, v=1) end end
Polyscope program is created.
A BeforeStart sequence is inserted where the script file is loaded into the main Polyscope program.
The function Flash_Out_1 is called which toggles Digital Output 1.
Then the function “Pause” is called with a parameter the determine the length of the pause.
Then the function “Calc” is called as a part of the variable “Result” assignment – which will insert the result from the returned variable in the function back into the variable “Result”.
Then the function “Move” is called that moves the robot to a position based on the parameters given in the function call – and the function calculate a new position value and the robot moves to this new position.
Then the function “If_Check” is called that moves the robot between two different position based on the status of the Digital Input 0.
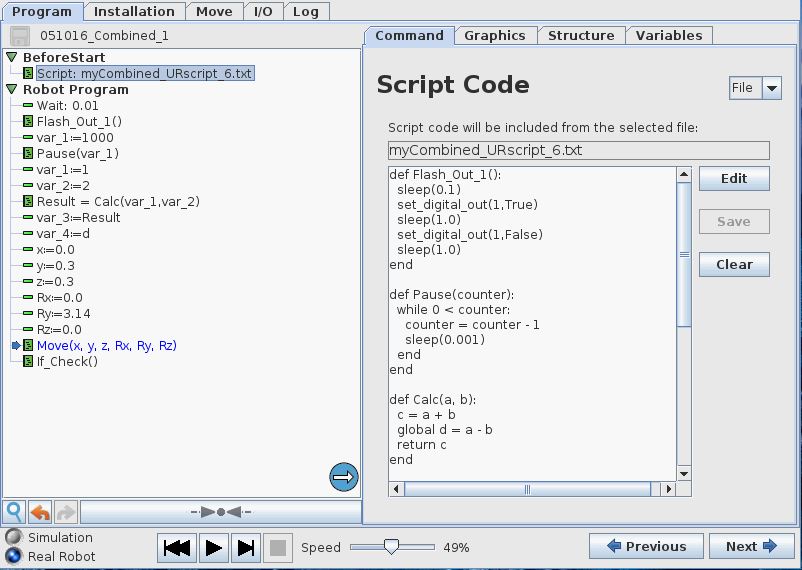
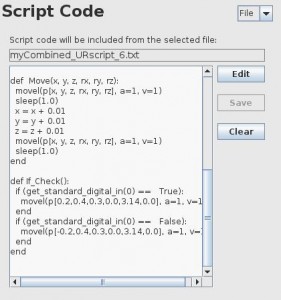
Program run:
Notice: var_1 change between 1000 and 1.
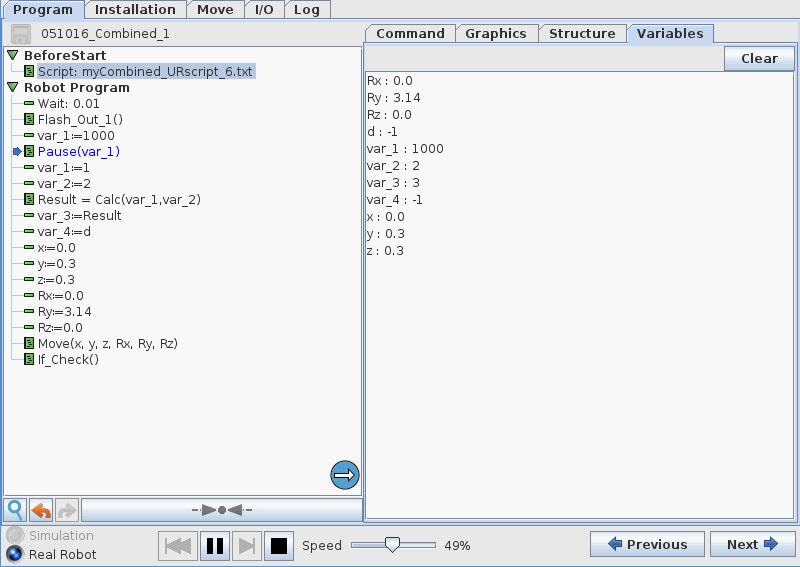
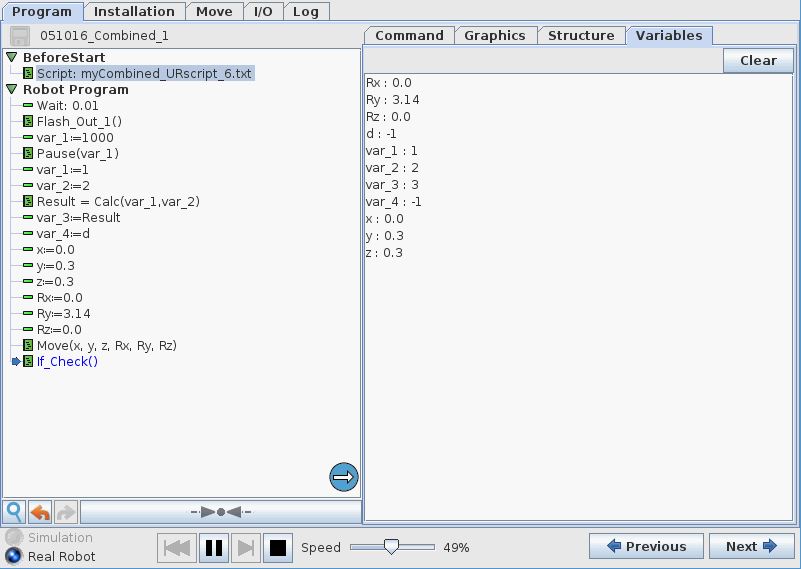
Example 7: Function with 2 lists of poses (Each list contain 6 poses i.e. a total of 12 poses).
A script file is create in Notepad on a PC. When the script file is embedded into the Polyscope and called from the main program the code in the script file will move the robot to 12 positions loaded into the script via the parameter transfer variables pose_x0 and pose_x1.
The pose_x0 variable contain 6 poses – (see polyscope program below).
The pose_x1 variable contain 6 poses – (see polyscope program below).
The while loop pick runs 6 times in order to pick each of the 6 poses in both variables. The first movel pick element number 0 from the variable pose_x0 and the robot moves there. A wait (sleep) makes sure the robot reaches the position before next command. The second movel pick element number 0 from the variable pose_x1 and the robot moves there. As the while loop runs 6 times all the 6 poses in both variables are picked one by one and therefore the robot moves to 12 positions.
The name of the function is “MoveLinear” and in this case it has an option of importing 2 variables as parameteres (pose_x0 and pose_x1).
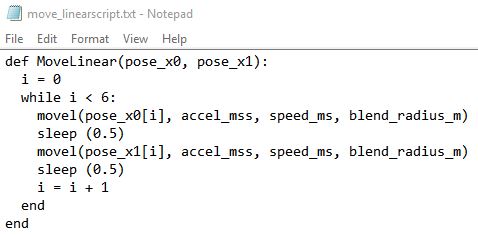
def MoveLinear(pose_x0, pose_x1): i = 0 while i < 6: movel(pose_x0[i], accel_mss, speed_ms, blend_radius_m) sleep (0.5) movel(pose_x1[i], accel_mss, speed_ms, blend_radius_m) sleep (0.5) i = i + 1 end end
The script code can be entered directly into the polyscope script editor as shown below – or imported from the file created externally in notepad.
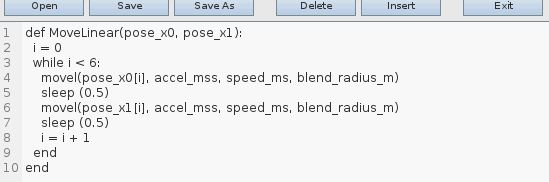
Polyscope program is created.
In the BeforeStart routine the script is entered into the polyscope environment.
In the main program the acceleration, speed and blend variables are created and given values. Also 12 poses are created which are the positions the robot needs to go to.
The 12 poses are inserted into two variables pose_x0_list and pose_x1_list which each contain a list on 6 poses.
As the name of the function in the script file is named “MoveLinear” the the script is called from the polyscope program with the command “MoveLinear(pose_x0_list, pose_x1_list)” which means the 2 variables pose_x0_list and pose_x1_list are transferred into the script routine.
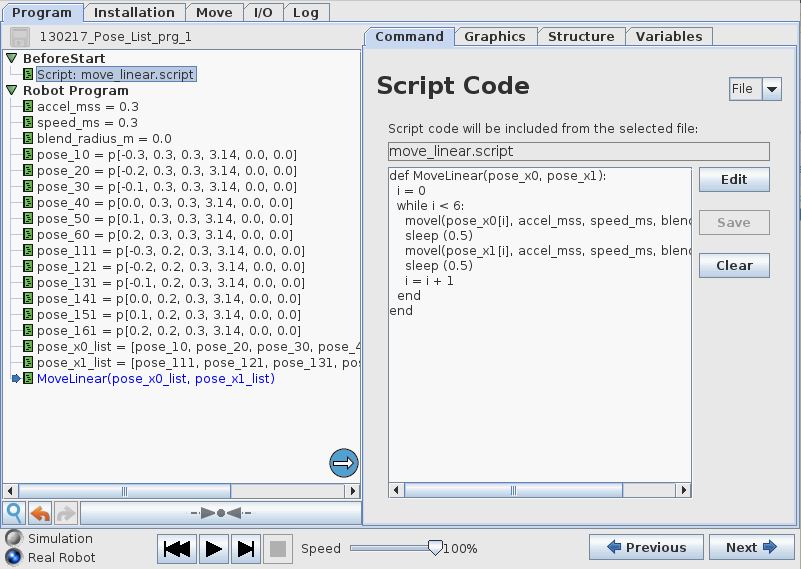
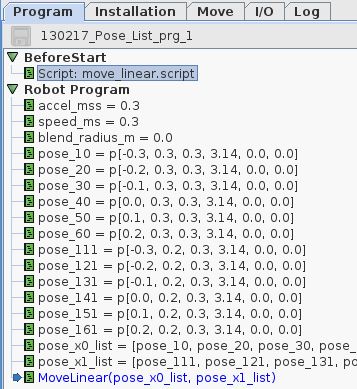
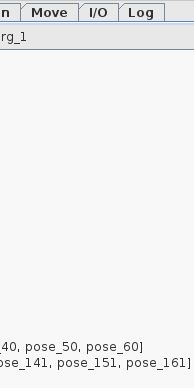
Program run:
The robot runs through the 12 positions.
Disclaimer: While the Zacobria Pte. Ltd. believes that information and guidance provided is correct, parties must rely upon their skill and judgement when making use of them. Zacobria Pte. Ltd. assumes no liability for loss or damage caused by error or omission, whether such an error or omission is the result of negligence or any other cause. Where reference is made to legislation it is not to be considered as legal advice. Any and all such liability is disclaimed.
If you need specific advice (for example, medical, legal, financial or risk management), please seek a professional who is licensed or knowledgeable in that area.
Author:
By Zacobria Lars Skovsgaard
Accredited 2015-2018 Universal Robots support Centre and Forum.